Introduction to Java
Java is a high-level, class-based, object-oriented programming language designed to have as few implementation dependencies as possible. It is a general-purpose language that is designed to be platform-independent, which means that Java programs can run on any device that has a Java Virtual Machine (JVM) installed.
Features of Java

Java has several key features that make it popular among developers:
- Platform Independence: Java code can run on any device that has a JVM.
- Object-Oriented: Everything in Java is treated as an object, which promotes modularity and reuse.
- Simple and Easy to Learn: Java's syntax is designed to be easy to understand and use.
- Robust: Java has strong memory management and exception handling capabilities.
- Secure: Java provides a secure environment with its built-in security features.
- Multithreaded: Java supports multithreading, which allows concurrent execution of programs.
Basic Syntax of Java
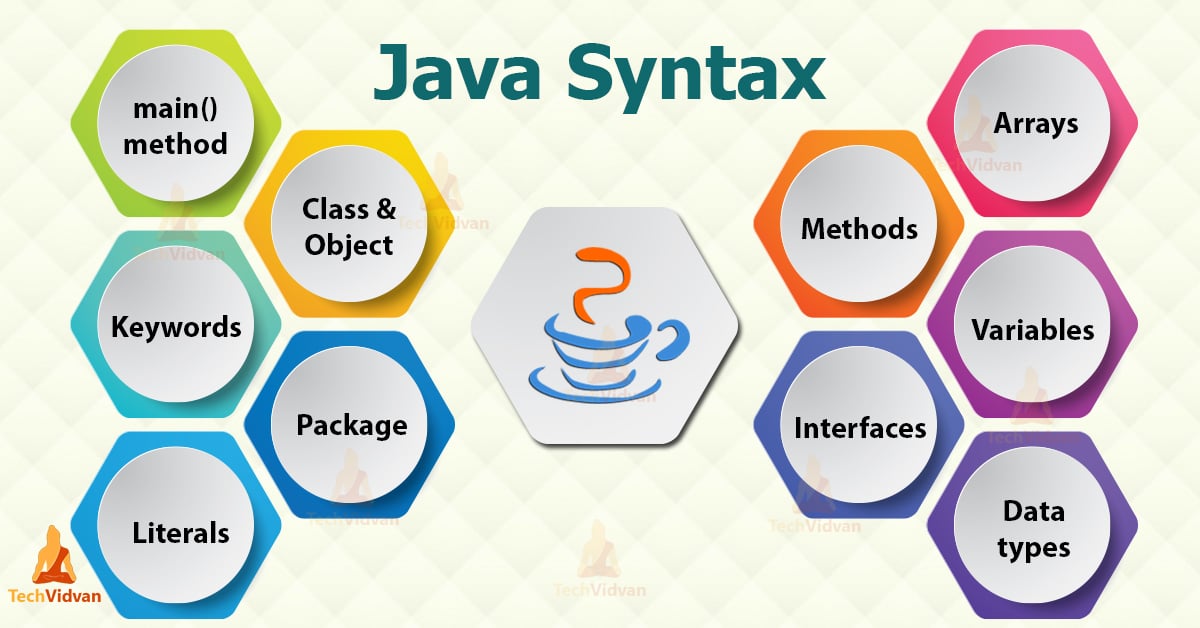
Java's syntax is similar to other C-based languages. Here are some basic components:
- Class: Java programs are organized into classes. A class is a blueprint for objects.
- Main Method: The entry point for any Java application is the `main` method.
- Statements: Java statements end with a semicolon (`;`).
- Comments: Java supports single-line (`//`) and multi-line (`/* */`) comments.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Object-Oriented Concepts
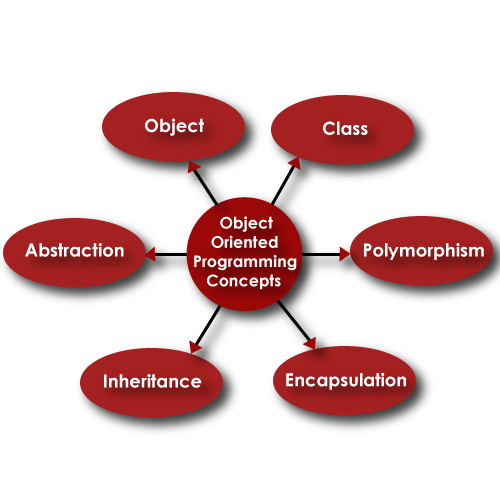
Java follows the object-oriented programming paradigm. Key concepts include:
- Encapsulation: Wrapping data and methods into a single unit called a class.
- Inheritance: Mechanism where a new class inherits properties and behaviors from an existing class.
- Polymorphism: Ability to perform a single action in different ways.
- Abstraction: Hiding complex implementation details and showing only the necessary features.
Use Cases of Java
Java is used in various domains, including:
- Web Development: Java is used to build server-side applications using technologies like Servlets and JSP.
- Mobile Development: Java is the primary language for Android development.
- Enterprise Applications: Java is used to build large-scale enterprise applications.
- Scientific Computing: Java is used for scientific and research applications due to its precision and reliability.